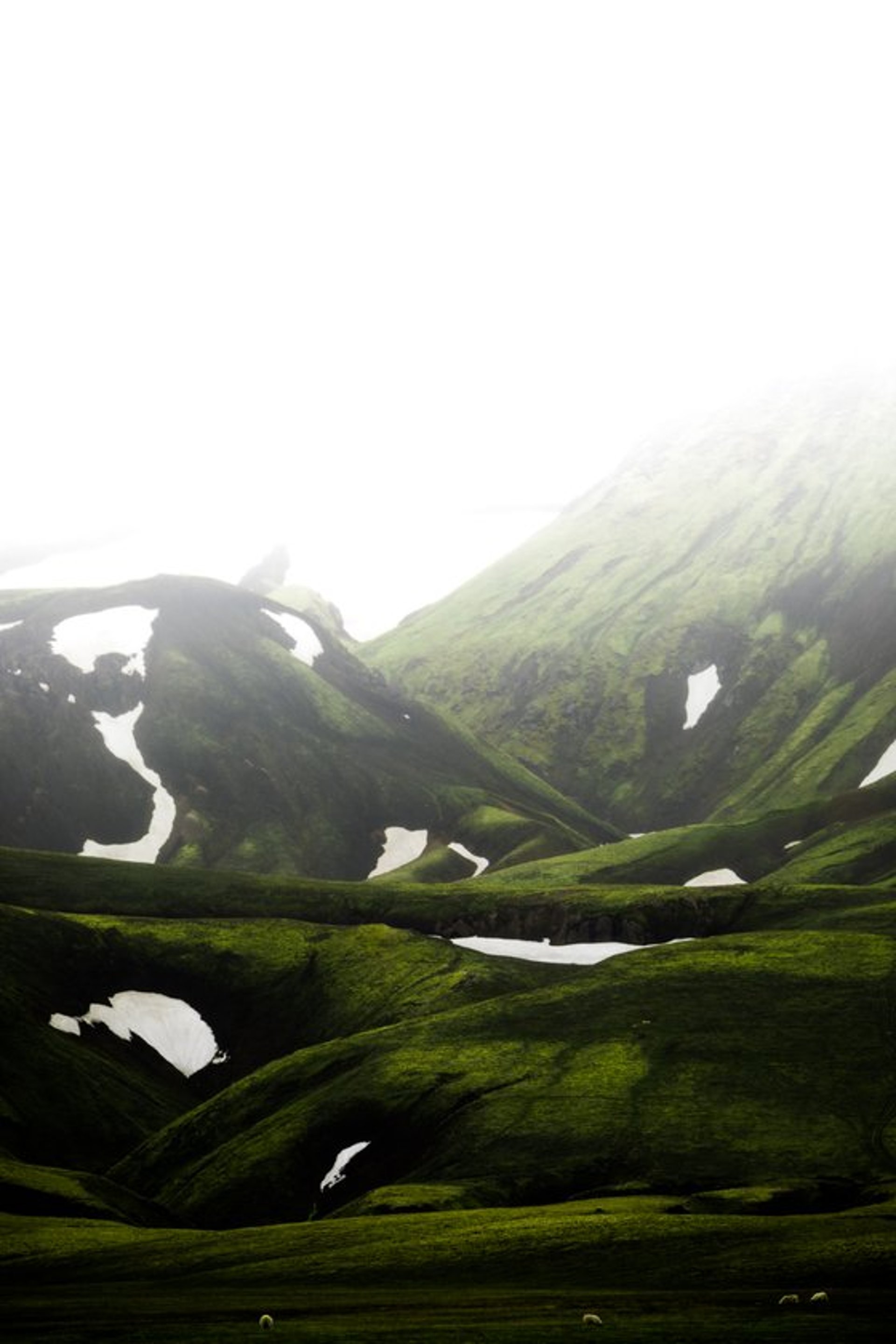
Understanding IF and Nested IF Statements in SQL with Examples
Master IF and Nested IF statements in SQL with real-world examples. Our comprehensive guide provides insights to enhance your query control. Elevate your SQL skills today!
In SQL, the IF statement is a powerful tool that allows you to control the flow of your queries based on certain conditions. It enables you to execute different sets of SQL statements based on whether a specified condition is true or false. Additionally, you can also nest IF statements within each other to create more complex conditional logic.
IF Statement Syntax
The basic syntax of the IF statement in SQL is as follows:
IF condition BEGIN -- SQL statements to be executed if the condition is true END
Here, "condition" represents the expression that evaluates to either true or false. If the condition is true, the SQL statements within the BEGIN and END block will be executed. If the condition is false, the block will be skipped, and the execution will continue with the next statement.
Examples of IF Statements in SQL
Let's explore some simple examples to understand how IF statements work in SQL.
Example 1: Checking if a Product Quantity is Sufficient
Suppose we have a table called "Products" with columns "ProductID" and "Quantity". We want to check if the quantity of a specific product is sufficient before allowing a purchase. If the quantity is greater than or equal to 10, the purchase is allowed; otherwise, it is denied.
IF (SELECT Quantity FROM Products WHERE ProductID = 123) >= 10 BEGIN PRINT 'Purchase allowed' END ELSE BEGIN PRINT 'Purchase denied' END
In this example, we use the IF statement to check the quantity of the product with ProductID 123. If the quantity is greater than or equal to 10, the message "Purchase allowed" will be printed. Otherwise, the message "Purchase denied" will be printed.
Example 2: Updating a Customer's Credit Limit
Let's consider a scenario where we need to update a customer's credit limit based on their payment history. If a customer has made more than three late payments, their credit limit should be reduced; otherwise, it remains unchanged.
DECLARE @LatePayments INT SELECT @LatePayments = COUNT(*) FROM Payments WHERE CustomerID = 456 AND PaymentStatus = 'Late' IF @LatePayments > 3 BEGIN UPDATE Customers SET CreditLimit = CreditLimit * 0.9 WHERE CustomerID = 456 END
In this example, we use the IF statement to count the number of late payments made by the customer with CustomerID 456. If the count is greater than 3, the customer's credit limit is reduced by 10% using the UPDATE statement.
Nested IF Statements
In addition to the basic IF statement, SQL also allows you to nest IF statements within each other. This enables you to create more complex conditional logic by evaluating multiple conditions.
Example: Determining a Student's Grade
Suppose we have a table called "Grades" with columns "StudentID" and "Score". We want to determine the grade of a student based on their score. The grading criteria are as follows:
- Score >= 90: Grade A
- Score >= 80: Grade B
- Score >= 70: Grade C
- Score >= 60: Grade D
- Score < 60: Grade F
DECLARE @Grade CHAR(1) SELECT @Grade = IF Score >= 90 THEN 'A' ELSE IF Score >= 80 THEN 'B' ELSE IF Score >= 70 THEN 'C' ELSE IF Score >= 60 THEN 'D' ELSE 'F' END END END END FROM Grades WHERE StudentID = 789
In this example, we use nested IF statements to determine the grade of the student with StudentID 789 based on their score. The result is stored in the variable @Grade, which will contain the appropriate grade based on the score.
Conclusion
The IF statement in SQL is a powerful tool that allows you to control the flow of your queries based on specified conditions. By using IF statements, you can execute different sets of SQL statements depending on whether a condition is true or false. Additionally, nesting IF statements within each other enables you to create more complex conditional logic.
Understanding how to use IF and nested IF statements in SQL will greatly enhance your ability to manipulate data and create dynamic queries. By leveraging the conditional logic provided by these statements, you can create more intelligent and efficient SQL code.