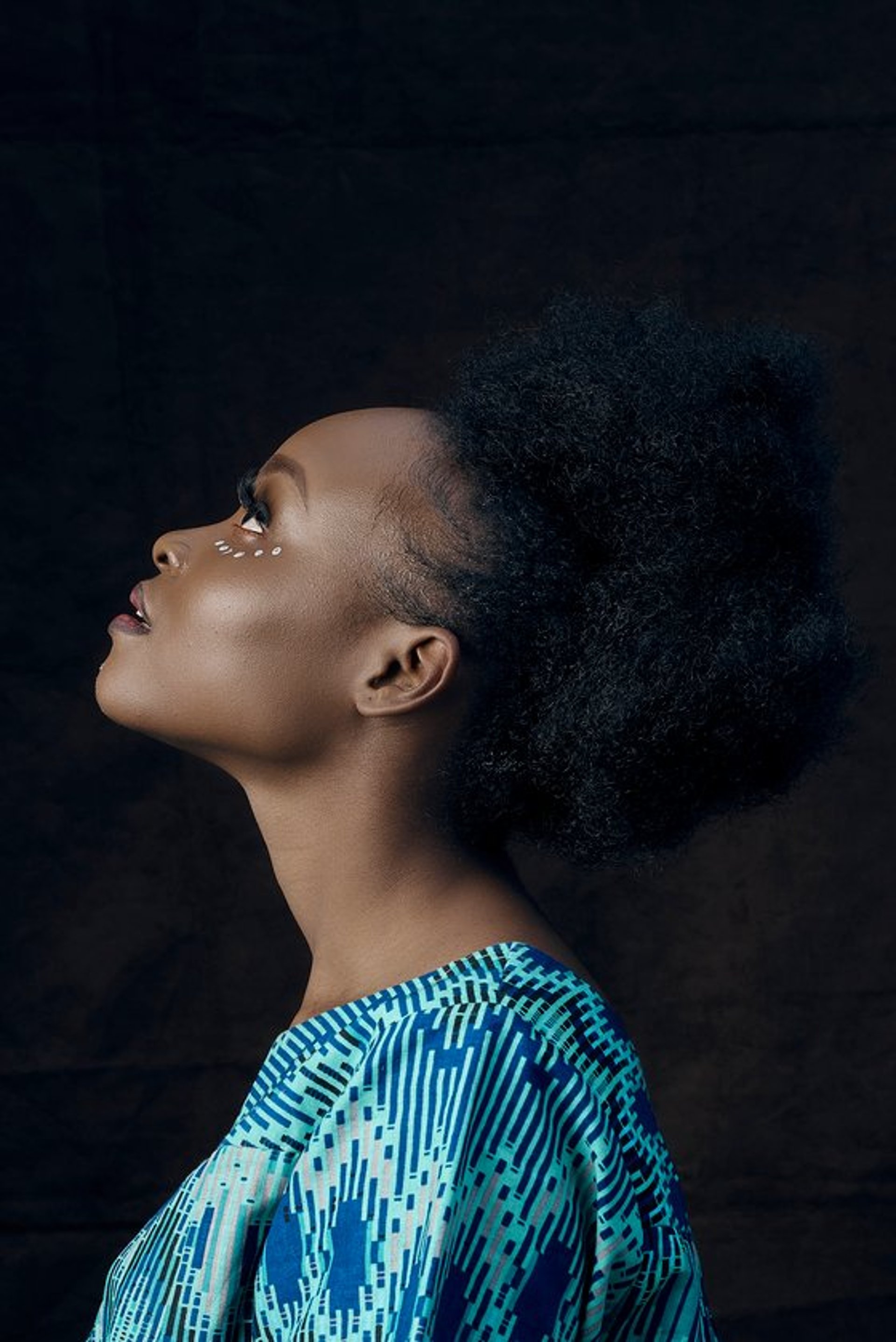
SQL Data Validation Techniques: Ensuring Data Integrity in Your Database
Ensure data integrity in your database with SQL data validation techniques. Explore effective methods for verifying and maintaining accurate data. Learn more!
When it comes to managing databases, data validation plays a crucial role in ensuring the accuracy, consistency, and integrity of the data. SQL, or Structured Query Language, provides various techniques for validating data to prevent errors and maintain the quality of your database. In this blog post, we will explore some SQL data validation techniques along with examples to help you implement effective data validation in your own database.
1. Check Constraints
Check constraints are a powerful feature in SQL that allow you to define rules for the values in a column. These constraints can be used to validate data based on specific conditions or expressions. Let's take a look at an example:
CREATE TABLE Employees (
EmployeeID INT,
FirstName VARCHAR(50),
LastName VARCHAR(50),
Age INT,
CONSTRAINT CHK_Age CHECK (Age >= 18)
);
In this example, we have a check constraint named "CHK_Age" that ensures the "Age" column only accepts values greater than or equal to 18. If any insert or update operation violates this constraint, an error will be thrown.
2. Unique Constraints
Unique constraints are used to enforce uniqueness on one or more columns in a table. They prevent duplicate values from being inserted into the specified columns. Let's consider an example:
CREATE TABLE Customers (
CustomerID INT,
Email VARCHAR(100),
CONSTRAINT UQ_Email UNIQUE (Email)
);
In this example, we have a unique constraint named "UQ_Email" that ensures the "Email" column only accepts unique values. If an attempt is made to insert a duplicate email address, an error will be thrown.
3. Foreign Key Constraints
Foreign key constraints are used to maintain referential integrity between tables. They ensure that values in a column (known as the foreign key) match values in another table (known as the primary key). Let's see an example:
CREATE TABLE Orders (
OrderID INT,
CustomerID INT,
OrderDate DATE,
CONSTRAINT FK_CustomerID FOREIGN KEY (CustomerID) REFERENCES Customers(CustomerID)
);
In this example, we have a foreign key constraint named "FK_CustomerID" that ensures the values in the "CustomerID" column of the "Orders" table match the values in the "CustomerID" column of the "Customers" table. If an attempt is made to insert a value that does not exist in the referenced table, an error will be thrown.
4. Data Type Constraints
Data type constraints are used to enforce the correct data type for a column. They ensure that the values inserted into the column match the specified data type. Let's take a look at an example:
CREATE TABLE Products (
ProductID INT,
ProductName VARCHAR(100),
Quantity INT,
CONSTRAINT CHK_Quantity CHECK (Quantity >= 0)
);
In this example, we have a check constraint named "CHK_Quantity" that ensures the "Quantity" column only accepts non-negative values. If an attempt is made to insert a negative value, an error will be thrown.
5. Regular Expressions
Regular expressions are a powerful tool for pattern matching and data validation. SQL provides various functions that allow you to use regular expressions to validate data. Let's consider an example:
SELECT *
FROM Customers
WHERE Email LIKE '%@example.com';
In this example, we are using the "LIKE" operator along with a regular expression to retrieve all customers whose email addresses end with "@example.com". This allows us to validate and filter data based on a specific pattern.
6. Stored Procedures
Stored procedures are a way to encapsulate SQL code and logic into reusable modules. They can be used to perform complex data validation tasks by implementing custom business rules. Let's see an example:
CREATE PROCEDURE InsertEmployee
@FirstName VARCHAR(50),
@LastName VARCHAR(50),
@Age INT
AS
BEGIN
IF @Age >= 18
BEGIN
INSERT INTO Employees (FirstName, LastName, Age)
VALUES (@FirstName, @LastName, @Age);
END
ELSE
BEGIN
RAISERROR('Age must be greater than or equal to 18.', 16, 1);
END
END;
In this example, we have a stored procedure named "InsertEmployee" that validates the age of the employee before inserting the data into the "Employees" table. If the age is less than 18, an error is raised.
Conclusion
Data validation is a critical aspect of managing databases effectively. By using SQL data validation techniques like check constraints, unique constraints, foreign key constraints, data type constraints, regular expressions, and stored procedures, you can ensure the accuracy, consistency, and integrity of your database. Implementing these techniques will help prevent errors, maintain data quality, and improve the overall reliability of your database system.
Remember, data validation is an ongoing process, and it's essential to regularly review and update your validation rules as your database evolves. By incorporating these techniques into your SQL development practices, you can build robust and reliable databases that meet the needs of your organization.