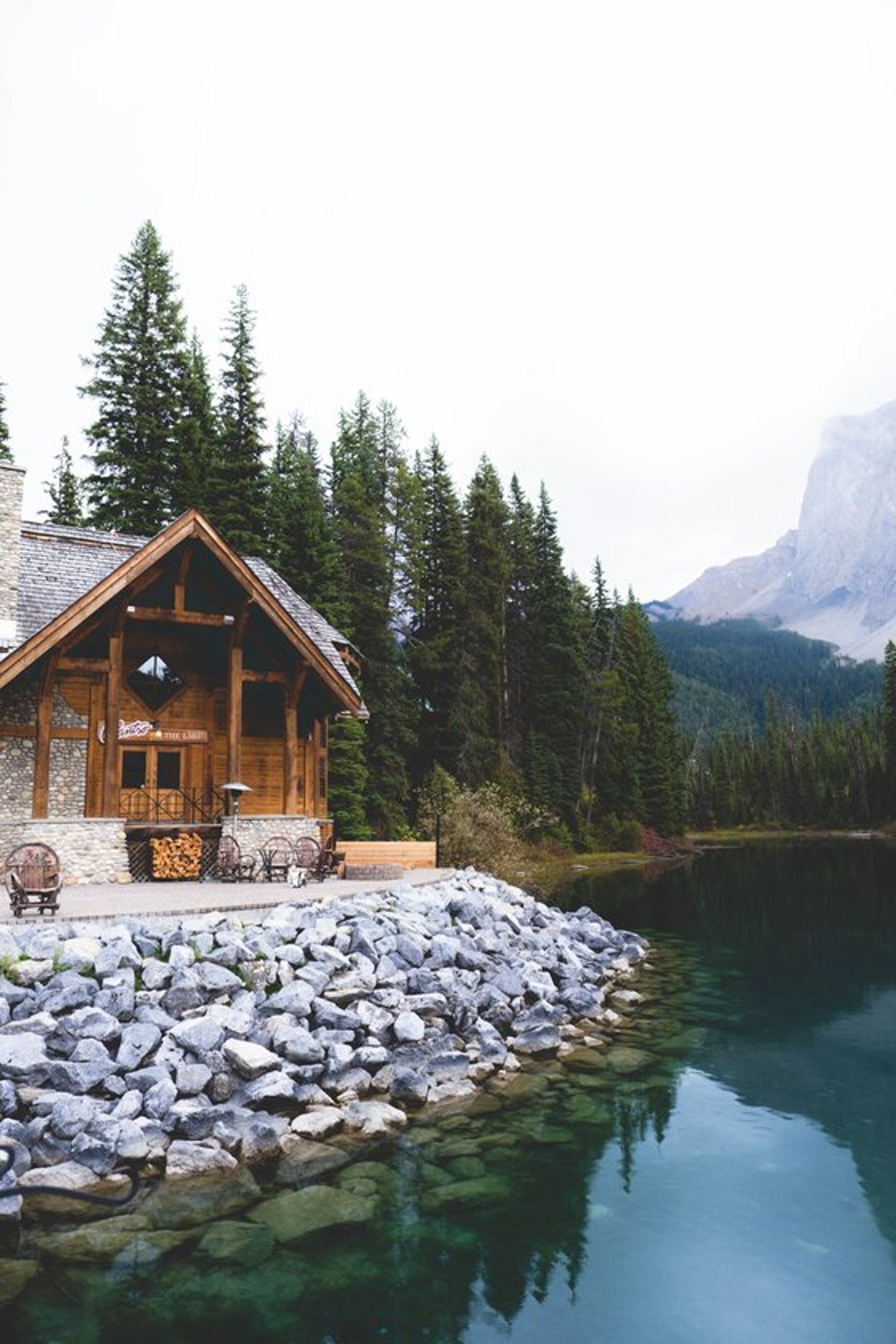
Advanced Stored Procedures in SQL Server: Unlocking the Power of Database Operations
Unlock the power of advanced stored procedures in SQL Server. Dive into our guide to harness the full potential of efficient and powerful database operations.
Stored procedures are a fundamental feature of SQL Server that allow you to encapsulate and execute complex database operations. They provide a way to organize and reuse code, improve performance, and enhance security. In this blog post, we will explore advanced techniques and examples of using stored procedures in SQL Server.
1. Parameterized Stored Procedures
Parameterized stored procedures enable you to pass input values to the procedure, making them more flexible and reusable. By using parameters, you can create dynamic queries and handle different scenarios based on user input.
Let's consider an example where we have a table called "Employees" with columns like "EmployeeID", "FirstName", and "LastName". We want to create a stored procedure that retrieves employee details based on the employee ID.
CREATE PROCEDURE GetEmployeeDetails
@EmployeeID INT
AS
BEGIN
SELECT * FROM Employees WHERE EmployeeID = @EmployeeID
END
In this example, the "@EmployeeID" parameter allows us to pass the employee ID as an argument when executing the stored procedure. This makes it easy to retrieve specific employee details without writing repetitive queries.
2. Error Handling and Transactions
Error handling and transactions are crucial aspects of database operations. Stored procedures provide a robust mechanism to handle errors and ensure data integrity through transaction management.
Let's consider a scenario where we need to insert data into two related tables, "Customers" and "Orders." We want to ensure that if an error occurs during the insertion of data into the "Orders" table, the transaction is rolled back, and no data is inserted.
CREATE PROCEDURE InsertCustomerWithOrder
@CustomerName NVARCHAR(100),
@OrderDescription NVARCHAR(200)
AS
BEGIN
BEGIN TRANSACTION
BEGIN TRY
INSERT INTO Customers (CustomerName) VALUES (@CustomerName)
DECLARE @CustomerID INT = SCOPE_IDENTITY()
INSERT INTO Orders (CustomerID, OrderDescription) VALUES (@CustomerID, @OrderDescription)
COMMIT TRANSACTION
END TRY
BEGIN CATCH
ROLLBACK TRANSACTION
-- Handle error or rethrow
END CATCH
END
In this example, we use the "BEGIN TRANSACTION" and "COMMIT TRANSACTION" statements to define a transaction block. If an error occurs within the block, the "ROLLBACK TRANSACTION" statement is executed, undoing any changes made within the transaction.
3. Dynamic SQL
Dynamic SQL allows you to construct and execute SQL statements dynamically at runtime. It provides a way to build queries based on varying conditions or user input.
Let's consider an example where we want to create a stored procedure that retrieves employee details based on different search criteria, such as employee ID, first name, or last name. We want the stored procedure to be flexible enough to handle any combination of search criteria.
CREATE PROCEDURE SearchEmployees
@EmployeeID INT = NULL,
@FirstName NVARCHAR(50) = NULL,
@LastName NVARCHAR(50) = NULL
AS
BEGIN
DECLARE @SQL NVARCHAR(MAX) = N'
SELECT * FROM Employees WHERE 1 = 1'
IF @EmployeeID IS NOT NULL
SET @SQL += N' AND EmployeeID = @EmployeeID'
IF @FirstName IS NOT NULL
SET @SQL += N' AND FirstName = @FirstName'
IF @LastName IS NOT NULL
SET @SQL += N' AND LastName = @LastName'
EXEC sp_executesql @SQL,
N'@EmployeeID INT, @FirstName NVARCHAR(50), @LastName NVARCHAR(50)',
@EmployeeID, @FirstName, @LastName
END
In this example, we use dynamic SQL to build the query based on the provided search criteria. The "sp_executesql" system stored procedure is used to execute the dynamically constructed SQL statement.
4. Table-Valued Parameters
Table-valued parameters (TVPs) allow you to pass sets of data as parameters to a stored procedure. This feature is particularly useful when you need to perform bulk operations or pass multiple rows of data.
Let's consider an example where we want to create a stored procedure that inserts multiple employee records into the "Employees" table using a TVP.
CREATE TYPE EmployeeType AS TABLE
(
EmployeeID INT,
FirstName NVARCHAR(50),
LastName NVARCHAR(50)
)
CREATE PROCEDURE InsertEmployees
@Employees EmployeeType READONLY
AS
BEGIN
INSERT INTO Employees (EmployeeID, FirstName, LastName)
SELECT EmployeeID, FirstName, LastName FROM @Employees
END
In this example, we define a user-defined table type called "EmployeeType," which represents the structure of the data we want to pass as a parameter. The "@Employees" parameter is of type "EmployeeType READONLY," allowing us to pass a table variable containing multiple employee records.
5. Recursive Stored Procedures
Recursive stored procedures enable you to perform recursive operations on hierarchical data structures. They are commonly used for tasks such as traversing tree-like structures or generating reports.
Let's consider an example where we have a table called "Categories" with columns like "CategoryID", "CategoryName", and "ParentCategoryID." We want to create a stored procedure that retrieves all the child categories for a given parent category.
CREATE PROCEDURE GetChildCategories
@ParentCategoryID INT
AS
BEGIN
WITH RecursiveCategories AS
(
SELECT CategoryID, CategoryName, ParentCategoryID
FROM Categories
WHERE ParentCategoryID = @ParentCategoryID
UNION ALL
SELECT C.CategoryID, C.CategoryName, C.ParentCategoryID
FROM Categories C
INNER JOIN RecursiveCategories RC ON C.ParentCategoryID = RC.CategoryID
)
SELECT CategoryID, CategoryName, ParentCategoryID
FROM RecursiveCategories
END
In this example, we use a common table expression (CTE) to define the recursive query. The CTE is then used in the SELECT statement to retrieve all the child categories for the specified parent category.
Conclusion
Stored procedures in SQL Server offer a wide range of advanced features and techniques that can greatly enhance the efficiency, flexibility, and security of your database operations. By leveraging parameterized stored procedures, error handling, dynamic SQL, table-valued parameters, and recursive procedures, you can unlock the full potential of SQL Server and optimize your database workflows.
Remember to use these advanced techniques judiciously and consider the specific requirements and constraints of your application. With the right understanding and implementation, advanced stored procedures can become powerful tools in your SQL Server arsenal.