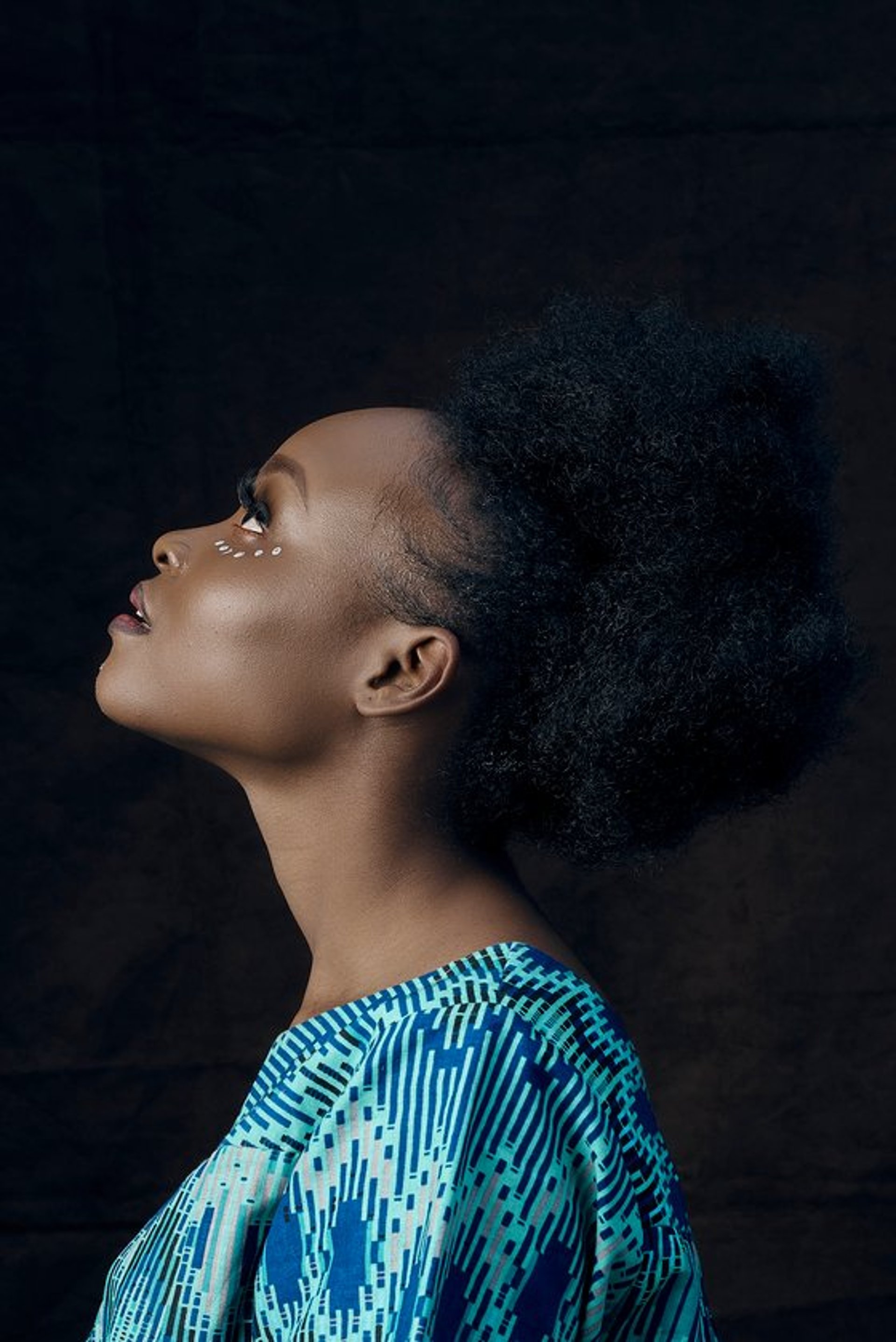
Understanding SQL Recursive Queries: A Comprehensive Guide with Examples
Master SQL recursive queries with our comprehensive guide and practical examples. Unlock the power of hierarchical data modeling for advanced database analysis.
SQL (Structured Query Language) is a powerful tool used to manage and manipulate databases. One of the most intriguing features of SQL is its ability to perform recursive queries. Recursive queries allow you to query data that references itself, making it possible to traverse hierarchical structures or solve complex problems that require iterative processing.
What are Recursive Queries?
In SQL, a recursive query is a query that refers to its own output. It enables you to perform operations on a dataset that has a recursive structure, such as a hierarchical relationship between rows. Recursive queries are particularly useful when dealing with data that has a parent-child relationship, like organizational charts, product categories, or even social networks.
Recursive queries are implemented using a combination of common table expressions (CTEs) and recursive anchor and recursive members. The anchor member is the base case, and the recursive member is the iterative step that builds upon the anchor member until the desired result is achieved.
Recursive Queries Syntax
The syntax for a recursive query in SQL typically follows this structure:
WITH RECURSIVE cte_name (column_list) AS ( -- Anchor member SELECT column_list FROM base_table UNION ALL -- Recursive member SELECT column_list FROM cte_name JOIN recursive_table ON join_condition ) SELECT * FROM cte_name;
Let's break down the different parts of this syntax:
- cte_name: The name of the common table expression that will be used throughout the query.
- column_list: The list of columns you want to select from the anchor and recursive members.
- base_table: The table or subquery that serves as the anchor member.
- recursive_table: The table or subquery that serves as the recursive member.
- join_condition: The condition that defines the relationship between the anchor and recursive members.
Example: Recursive Query for Hierarchical Data
Let's consider an example where we have a table called employees
that stores information about employees and their managers. The table has two columns: employee_id
and manager_id
. The manager_id
column refers to the employee_id
of the employee's manager.
Our goal is to retrieve a hierarchical structure of employees and their managers, starting from a specific employee. We can achieve this using a recursive query.
CREATE TABLE employees ( employee_id INT, manager_id INT ); INSERT INTO employees VALUES (1, NULL); INSERT INTO employees VALUES (2, 1); INSERT INTO employees VALUES (3, 1); INSERT INTO employees VALUES (4, 2); INSERT INTO employees VALUES (5, 2); INSERT INTO employees VALUES (6, 3); INSERT INTO employees VALUES (7, 3);
Now, let's write a recursive query to retrieve the hierarchical structure:
WITH RECURSIVE employee_hierarchy (employee_id, manager_id, level) AS ( -- Anchor member SELECT employee_id, manager_id, 0 FROM employees WHERE employee_id = 1 UNION ALL -- Recursive member SELECT employees.employee_id, employees.manager_id, employee_hierarchy.level + 1 FROM employees JOIN employee_hierarchy ON employees.manager_id = employee_hierarchy.employee_id ) SELECT employee_id, manager_id, level FROM employee_hierarchy;
In this example, we start with the anchor member, which selects the employee with an employee_id
of 1. Then, in the recursive member, we join the employees
table with the employee_hierarchy
CTE, using the manager_id
and employee_id
columns, respectively. We also increment the level
by 1 to keep track of the hierarchy depth.
The result of this query will be:
employee_id | manager_id | level ------------+------------+------ 1 | | 0 2 | 1 | 1 3 | 1 | 1 4 | 2 | 2 5 | 2 | 2 6 | 3 | 2 7 | 3 | 2
As you can see, the query retrieves the hierarchical structure of employees and their managers, starting from the employee with an employee_id
of 1.
Use Cases for Recursive Queries
Recursive queries can be applied to various scenarios where data has a recursive or hierarchical structure. Here are a few common use cases:
1. Organization Charts
Recursive queries are commonly used to represent and query organizational charts. By storing the relationships between employees and their managers, you can easily retrieve the hierarchical structure of an organization, including the reporting lines and levels of management.
2. Product Categories
In an e-commerce system, products are often organized into categories and subcategories. Recursive queries can be used to retrieve the entire category hierarchy, making it easier to navigate and analyze product data.
3. Social Networks
Recursive queries can also be applied to social networks, where users are connected to each other through friend relationships. By using recursive queries, you can traverse the network and retrieve connections, friends of friends, or even find the shortest path between two users.
Conclusion
Recursive queries in SQL provide a powerful mechanism for querying hierarchical or recursive data structures. By leveraging common table expressions and recursive members, you can easily traverse and analyze complex relationships within your database. Understanding how to write and use recursive queries opens up new possibilities for solving problems that require iterative processing.
Remember, recursive queries should be used judiciously, as they can be resource-intensive and may require careful optimization for large datasets. However, when used appropriately, recursive queries can greatly simplify the retrieval and manipulation of hierarchical data.